Insertion Sort Algorithmus in Java mit Programmbeispiel
Was ist der Insertion Sort Algorithmus?
Insertion Sort ist ein einfacher Sortieralgorithmus, der für kleine Datensätze geeignet ist. Während jeder Iteration wird der Algorithmus:
- Entfernt ein Element aus einem Array.
- Vergleicht es mit dem größten Wert im Array.
- Bewegt das Element an die richtige Stelle.
Einfügungssortieralgorithmus-Prozess
So funktioniert der Einfüge-Sortieralgorithmus grafisch:
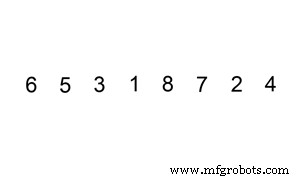
Einfüge-Sortieralgorithmus-Prozess
Java-Programmbeispiel zum Sortieren eines Arrays mit Insertion Sort Algorithm:
package com.guru99; public class InsertionSortExample { public static void main(String a[]) { int[] myArray = {860,8,200,9}; System.out.println("Before Insertion Sort"); printArray(myArray); insertionSort(myArray);//sorting array using insertion sort System.out.println("After Insertion Sort"); printArray(myArray); } public static void insertionSort(int arr[]) { int n = arr.length; for (int i = 1; i < n; i++) { System.out.println("Sort Pass Number "+(i)); int key = arr[i]; int j = i-1; while ( (j > -1) && ( arr [j] > key ) ) { System.out.println("Comparing "+ key + " and " + arr [j]); arr [j+1] = arr [j]; j--; } arr[j+1] = key; System.out.println("Swapping Elements: New Array After Swap"); printArray(arr); } } static void printArray(int[] array){ for(int i=0; i < array.length; i++) { System.out.print(array[i] + " "); } System.out.println(); } }
Codeausgabe:
Before Insertion Sort 860 8 200 9 Sort Pass Number 1 Comparing 8 and 860 Swapping Elements: New Array After Swap 8 860 200 9 Sort Pass Number 2 Comparing 200 and 860 Swapping Elements: New Array After Swap 8 200 860 9 Sort Pass Number 3 Comparing 9 and 860 Comparing 9 and 200 Swapping Elements: New Array After Swap 8 9 200 860 After Insertion Sort 8 9 200 860
Java
- calloc()-Funktion in der C-Bibliothek mit Programm BEISPIEL
- Java Hallo Welt:So schreiben Sie Ihr erstes Java-Programm mit Beispiel
- Kapselung in Java OOPs mit Beispiel
- String Length() Methode in Java:So finden Sie mit Beispiel
- Java String charAt() Methode mit Beispiel
- Java-String enthält()-Methode | Überprüfen Sie die Teilzeichenfolge mit Beispiel
- Java-String-EndsWith()-Methode mit Beispiel
- Java BufferedReader:Lesen von Dateien in Java mit Beispiel
- Bubble-Sort-Algorithmus in Java:Array-Sortierprogramm &Beispiel
- Auswahlsortierung im Java-Programm mit Beispiel